Android write to file

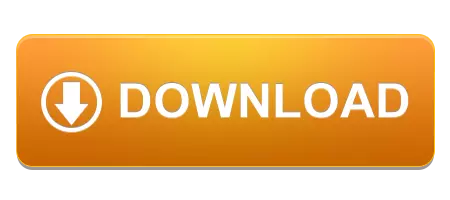

Reading lines from a file Now we have some data information writting to the myfilename. However, this will change in a future release. Write to the file. Using Internal Storage Internal storage refers to the on device hard drive and does not include SD cards. Note: You aren't required to check the amount of available space before you save your file. The most straightforward way to delete a file is to have the opened file reference call delete() on itself. This is the reason why strokes was declared as transient. Private files Files that rightfully belong to your app and should be deleted when the user uninstalls your app. A File object is suited to reading or writing large amounts of data in start-to-finish order without skipping around. Your application always has permission to read and write files in its internal storage directory. There are some difference from res directory. External storage: It's not always available, because the user can mount the external storage as USB storage and in some cases remove it from the device. It's world-readable, so files saved here may be read outside of your control. None of that applies to assets. If the returned state is equal to MEDIA_MOUNTED. Any application can access, overwrite, or delete files stored on the external storage. However, you should manually delete all cached files created with getCacheDir() on a regular basis and also regularly delete other files you no longer need. Finally, the names of the resources are turned into constant field names that are checked at compile time, so there's less of an opportunity for mismatches between the code and the resources themselves. This class is used to store information in a file. External storage is the best place for files that don't require access restrictions and for files that you want to share with other apps or allow the user to access with a computer. The content from the mySettings variable should now be writting to the file myfilename. Files saved here are accessible by only your app by default. In general, what I understand is that to write a non-serializable object to file we need to make it transient and define our readObject and writeObject methods. MEDIA_MOUNTED_READ_ONLY then you can only safely read. This returns the root directory for your app's private directory on the external storage. This could be done like this: We have now read the content of the myfilename. Users appreciate this option when the APK size is very large and they have an external storage space that's larger than the internal storage. I'll go back and fix this as soon I have a chance. Choose Internal or External Storage All Android devices have two file storage areas: "internal" and "external" storage. For more information, see App Install Location. You can instead try writing the file right away, then catch an IOException if one occurs. These methods provide the current available space and the total space in the storage volume, respectively. Why not just write the settings to a file and then read the file content when the application starts up. For example, the following methods are useful to determine the storage availability: Although the external storage is modifiable by the user and other apps, there are two categories of files you might save here: Public files Files that should be freely available to other apps and to the user. Technically, another app can read your internal files if you set the file mode to be readable. For instance, files saved in DIRECTORY_RINGTONES are categorized by the system media scanner as ringtones instead of music. In order to write data to SDCard, the application need permission WRITE_EXTERNAL_STORAGE. This information is also useful to avoid filling the storage volume above a certain threshold. It’s time to continue our sub-series on persistence as part of the 31 Days of Android. These names come from the early days of Android, when most devices offered built-in non-volatile memory (internal storage), plus a removable storage medium such as a micro SD card (external storage). You can access them by calling the getCacheDir and getExternalCacheDir methods, respectively, from the current Context. The getExternalStoragePublicDirectory method accepts a string parameter that determines which subdirectory you want to access using a series of Environment static constants: DIRECTORY_DCIM - pictures and videos taken by the device DIRECTORY_DOWNLOADS - files downloaded by the user DIRECTORY_MOVIES — movies DIRECTORY_MUSIC — audio fi les that represent music DIRECTORY_PICTURES — pictures Note that if the returned directory doesn’t exit, you must create it before writing fi les to the directory, as shown in the following snippet Online video Guys from Webucator make nice video about How to Read and Write Files in Android. For example, here's how to write some text to a file: Or, if you need to cache some files, you should instead use createTempFile(). However, if your app uses the WRITE_EXTERNAL_STORAGE permission, then it implicitly has permission to read the external storage as well. Once you’ve copied that file to your local computer and open it, you’ll see that whatever you had in the EditText when you tapped the button made it into the file. When the user uninstalls your app, the system removes all your app's files from internal storage. You don’t need any permissions to save files on the internal storage. Next, prior to trying to access the external storage, you need to check its state using the Environment. If the system begins running low on storage, it may delete your cache files without warning. To read more about this Android check out these books. SD Card) or an internal non-removable storage that is accessed in the same manner. The most important thing to remember when storing files on external storage is that no security is enforced on files stored here. Although these files are technically accessible by the user and other apps because they are on the external storage, they are files that realistically don't provide value to the user outside your app. Create the main layout as activity_main and main Activity as MainActivity. For example: Alternatively, you can call openFileOutput() to get a FileOutputStream that writes to a file in your internal directory. The following lists summarize the facts about each storage space. This lesson describes how to work with the Android file system to read and write files with the File APIs. It is only a pointer to the file on your hard drive (or other storage medium, like an SSD, USB drive, network share). In this tutorial we are going to Write data in text file in internal SD card and Read data from a text file from internal SD card. Also, many parts of the API support the use of resource identifiers. These directory names ensure that the files are treated properly by the system. For example, additional resources downloaded by your app or temporary media files. For example, if you change the file's encoding before you save it by converting a PNG image to JPEG, you won't know the file's size beforehand. To overwrite a file we need to make our own ObjectOutputStream. We check if the file size is greater that zero, and according to this we create our OutputStream. When the user uninstalls your app, these files should remain available to the user. If the file is saved on internal storage, you can also ask the Context to locate and delete a file by calling deleteFile() : Note: When the user uninstalls your app, the Android system deletes the following: All files you saved on internal storage All files you saved on external storage using getExternalFilesDir(). The second parameter to openFileOutput is the operating mode. This is particularly useful for applications that provide functionality that replaces or augments system applications, such as the camera, that store files in standard locations. Other apps cannot browse your internal directories and do not have read or write access unless you explicitly set the files to be readable or writable. With resources, there's built-in support for providing alternatives for different languages, OS versions, screen orientations, etc. DIRECTORY_MUSIC : Similarly to the pre 2. Also you can try online course Android Training. None of that is available with assets. To create a new file in one of these directories, you can use the File() constructor, passing the File provided by one of the above methods that specifies your internal storage directory. Tip: Although apps are installed onto the internal storage by default, you can specify the android:installLocation attribute in your manifest so your app may be installed on external storage. Some devices divide the permanent storage space into "internal" and "external" partitions, so even without a removable storage medium, there are always two storage spaces and the API behavior is the same whether the external storage is removable or not. Remember that getExternalFilesDir() creates a directory inside a directory that is deleted when the user uninstalls your app. To read the information from the file, we need to the InputReader, InputReaderStream and BufferedReader, so just import them like this: All we have to do now, is open the myfilename. Reading from the Local File Now that you’ve handled writing to the local file, it’s a small step to reading from that file. When the user uninstalls your app, the system removes your app's files from here only if you save them in the directory from getExternalFilesDir(). Save a File on Internal Storage When saving a file to internal storage, you can acquire the appropriate directory as a File by calling one of two methods: getFilesDir() Returns a File representing an internal directory for your app. Could you tell me if it is OK or how could it be improved? Query Free Space If you know ahead of time how much data you're saving, you can find out whether sufficient space is available without causing an IOException by calling getFreeSpace() or getTotalSpace(). SD Card) or an internal non-removable storage that is accessed in the same manner. MEDIA_MOUNTED then you should be safe to read and write. Be sure to delete each file once it is no longer needed and implement a reasonable size limit for the amount of memory you use at any given time, such as 1MB. Using External Storage External storage is typically either a removable storage media (i. You may need to do this if you don't know exactly how much space you need. The returned location is where users will typically place and manage their own files of each type. The method takes an argument specifying the type of file you want to save so that they can be logically organized with other public files, such as DIRECTORY_MUSIC or DIRECTORY_PICTURES. For example, the following method extracts the file name from a URL and creates a file with that name in your app's internal cache directory: Note: Your app's internal storage directory is specified by your app's package name in a special location of the Android file system. However, the other app would also need to know your app package name and file names. You can query the external storage state by calling getExternalStorageState(). We can then add some code, to handle the file writing: There you go. However, the system does not guarantee that you can write as many bytes as are indicated by getFreeSpace(). This simulates an InputStream, which you can pass to the most of the classes. For example, photos captured by your app or other downloaded files. So as long as you use MODE_PRIVATE for your files on the internal storage, they are never accessible to other apps. DIRECTORY_MUSIC if you want to put files in. Point is not serializable. Delete a File You should always delete files that you no longer need. Regardless of whether you use getExternalStoragePublicDirectory() for files that are shared or getExternalFilesDir() for files that are private to your app, it's important that you use directory names provided by API constants like DIRECTORY_PICTURES. To ensure that your app continues to work as expected, you should declare this permission now, before the change takes effect. Internal storage gives you the ability to prevent other applications from accessing the files you save and are tied directly to your app. Internal storage: It's always available. Pass in the filename (without the extension) as the variable name from the R. For example, it's good for image files or anything exchanged over a network. When the user uninstalls your app, the system deletes all files in your app's external private directory. You have to write the file using some classes in the Java API Since you said you wanted to keep everything in memory and don't want to write anything, you might try to use ByteArrayInputStream. Internal storage is best when you want to be sure that neither the user nor other apps can access your files. For example, here's a method you can use to create a directory for an individual photo album: If none of the pre-defined sub-directory names suit your files, you can instead call getExternalFilesDir() and pass null. This lesson shows how to perform basic file-related tasks in your app. Obtain Permissions for External Storage To write to the external storage, you must request the WRITE_EXTERNAL_STORAGE permission in your manifest file : Caution: Currently, all apps have the ability to read the external storage without a special permission. If your app needs to read the external storage (but not write to it), then you will need to declare the READ_EXTERNAL_STORAGE permission. So I think what you want is writing it to the hard drive. Files stored in either cache location will be erased when the application is uninstalled. Creating Project Create a new Android Application project in eclipse with package as learn2crack. Otherwise, you probably shouldn't write to storage. For example: If you want to save files that are private to your app, you can acquire the appropriate directory by calling getExternalFilesDir() and passing it a name indicating the type of directory you'd like. If you want to save public files on the external storage, use the getExternalStoragePublicDirectory() method to get a File representing the appropriate directory on the external storage. Writing Shared Files to External Storage Much like when you call getExternalFilesDir and you can pass in a type, there are public folders on external storage that have specific types. Each directory created this way is added to a parent directory that encapsulates all your app's external storage files, which the system deletes when the user uninstalls your app. How the settings come from the application to the mySettings variable is another subject and is not covered here. To make sure that we are able to overwrite file, we need to create our own ObjectOutputStream. To access these read-only file resources, call the openRawResource method from your application’s Resource object to receive an InputStream based on the specified file. To do this we need the OutputStream and OutputStreamReader, so lets import them like this: Lets say the application has a save button somewhere and when this is clicked the onClick(View) is called. There are three steps: Open the file with Context. Files stored in the internal cache will potentially be erased by the system when it is running low on available storage; files stored on the external cache will not be erased, as the system does not track available storage on external media.
Other topics:
- Wifi software for android tablet
- Top 100 games for android free download
- Android 4 firmware download
- Sony playstation 1 games
- Best android games 2015